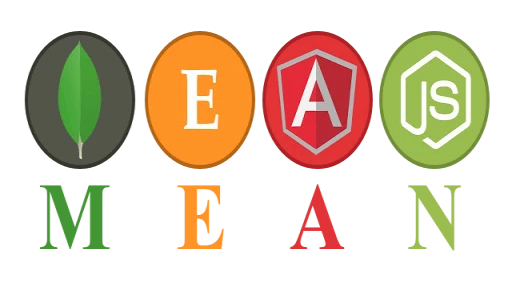
Single Page MEAN Application Setup : 10 Mintues
MongoDB
Express
Angular
NodeJS
Application
Setup
- By Code solution
- Jan 20th, 2021
- 0 comments
- 0
In today's tutorial, we will be looking at the starting setup for a Node.js, AngularJS, MongoDB, and Express application (otherwise known as MEAN).
Application Requirements
- Single Page Application
- Node.js backend with Express and MongoDB
- AngularJS frontend
Application Structure
--app #frontend code angularjs #angularjs libary js controller about.js contact.js home.js app.js pages header.html home.html about.html contact.html index.html --node_modules #npm package package-lock.json package.json server.js # backend Code
Creating a directory:
To start with, create an empty directory named MEANJS:
mkdir MEANJS
then move inside the newly created directory:
cd MEANJS
Then Run initialize the package.json by running Command:
npm init
We also need to install Socket.io, which is the main dependency of our project, ExpressJS,Http.
npm install --save express morgan mongoose body-parser method-override multer fs
Creating a server: the code
Create a file named server.js inside your project’s directory. This will hold the actual server
var express = require('express'); var app = express(); var morgan = require('morgan'); var mongoose = require('mongoose'); var bodyParser = require('body-parser'); var methodOverride = require('method-override'); var multer = require('multer'); var fs = require("fs"); //allow cross origin requests app.use(function(req, res, next) { res.setHeader("Access-Control-Allow-Origin", "*"); res.header("Access-Control-Allow-Methods", "POST, PUT, OPTIONS, DELETE, GET"); res.header("Access-Control-Max-Age", "3600"); res.header("Access-Control-Allow-Headers", "Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With"); next(); }); // configuration app.use(express.static(__dirname + '/app')); // set the static files location /app/img will be /img for users app.use('/app/uploads',express.static(__dirname + '/app/uploads')); app.use(morgan('dev')); app.use(bodyParser.json({limit: '50mb'})); // log every request to the console app.use(bodyParser.urlencoded({limit: '50mb','extended':'true'})); // parse application/x-www-form-urlencoded // parse application/json app.use(bodyParser.json({ type: 'application/vnd.api+json' })); // parse application/vnd.api+json as json // connect to mongoDB database mongoose.connect('mongodb://localhost/meanjs'); var Schema = mongoose.Schema; // user schema var userSchema = new Schema({ user_name: String, password: String, first_name: String, last_name: String, user_email:String, phone: Number, status: String, date: { type: Date, default: Date.now } }); var User = mongoose.model('User', userSchema); module.exports = User; // create user app.post('/api/createUser', function(req, res) { User.findOne({ 'user_email' : req.body.user_email }, function(err, user) { // if there are any errors, return the error if (err){ return res.send(err); } // check to see if there already a user with that email if (user) { return res.json({"message":"Email already exist"}); } else { User.create(userobj, function(err, user) { if (err){ res.send(err); } if(user) { res.json(user); } }); } }); }); // update user app.put('/api/updateUser', function(req, res) { User.findByIdAndUpdate(req.body._id, req.body, {new: true}, function(err, user) { if (err){ res.send(err); } res.json(user); }); }); // delete user app.delete('/api/removeUser', function(req, res) { User.remove({ _id : req.body._id }, function(err, user) { if (err) res.send(err); res.json(user); }); }); // get users app.post('/api/getUsers', function(req, res) { User.find(req.body,function(err, users) { if (err) res.send(err) res.json(users); }); }); app.listen(process.env.PORT || 9000, function(){console.log("App listening on port 9000");});
Save the above code and run it with the following command.
node server.js
Output :
After creating server-side code then please click this link for to frontend side :
http://codesolution.co.in/detail/post/angularjs-nested-routing--using-ui-routing---10-minutes
Thanks : )
Github Link: https://github.com/Sudarshan101/Meanjs