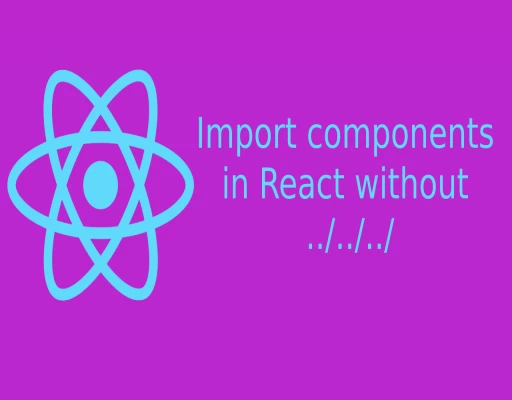
Import components in ReactJS without ../../../
Import
components
ReactJS
- By Code solution
- Aug 24th, 2021
- 0 comments
- 5
Steps to configure absolute Import in Create React App without any third-party packages. Are you importing components like ../../../../components
? Then you should update to Absolute imports.
Benefits of Absolute Import
- You can move your existing code to other components with imports without any changes.
- You can easily identify that where the component is actually placed using the import path.
- Cleaner Code.
- Easier to write.
Configure Absolute Import
To support absolute import create a file name jsconfig.json
in your root directory and add the below code.
{
"compilerOptions": {
"baseUrl": "src"
},
"include": ["src"]
}
Now let's convert the relative imports in the below component to Absolute Import
import React from 'react'; import Button from '../../components/Button'; import { green } from '../../utils/constants/colors'; function SuccessButton(){ return <Button color={green} />; } export default SuccessButton;
The Above imports will be changed to as below:
import React from 'react'; import Button from 'components/Button'; import { green } from 'utils/constants/colors'; function SuccessButton(){ return <Button color={green} />; } export default SuccessButton;
Now our imports are clean and understandable.